Git Commands Cheat Sheet
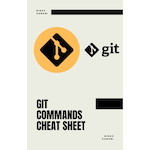
Buy PDF
Here are some of the most common and useful Git commands
Git Configuration
Command |
Description |
$ git config |
π οΈ Get and set configuration variables for Git. |
$ git config --global user.name "User name" |
βοΈ Set the name. |
$ git config --global user.email "your-email@example.com" |
βοΈ Set the email. |
$ git config --global core.editor "your-editor" |
π Set the default editor. |
$ git config --global color.ui auto |
π Turns on color output for Git commands. |
$ git config --list |
βΉοΈ See all your settings. |
Create Project
Command |
Description |
$ git init |
π Create a local repository. |
$ git clone |
π Clone a remote repository. |
Local Changes
Command |
Description |
$ git add file-path |
β Adds a file to the staging area. |
$ git add . |
β Add all files to the staging area. |
$ git commit -m " Commit Message" |
πΎ Commit file permanently in the version history. |
Track Changes
Command |
Description |
$ git diff |
π Shows the differences between your folder and the stage. |
$ git diff --staged |
π Shows the differences between the stage and the last commit. |
$ git diff HEAD |
π Track the changes after committing a file. |
$ git diff <branch-2> |
π Track the changes between two commits. |
$ git status |
βΉοΈ Display the state of the working directory and the staging area. |
$ git show |
βΉοΈ Show the newest commit on a branch. |
$ git diff -- file-path |
π Show changes for a particular file. |
$ git diff branch-1 branch-2 --name-only |
π Show difference between two branches (file names only). |
$ git diff branch-1 branch-2 -- file-path |
π Show differences between two branches for a specific file. |
Commit History
Command |
Description |
$ git log |
π Display the most recent commits and the status of the head. |
$ git log -oneline |
π Display the output as one commit per line. |
$ git log -stat |
π Displays the files that have been modified. |
$ git log -p |
π Display the modified files with location. |
Ignoring files
Command |
Description |
$ touch .gitignore |
π Create a file named .gitignore to list ignored files. |
$ git update-index --skip-worktree <file_name> |
π Mark a file as βskip-worktreeβ to ignore changes in it. |
$ git update-index --no-skip-worktree <file_name> |
π Unmark a file as βskip-worktreeβ to stop ignoring changes in it. |
A collection of useful .gitignore
templates for different languages or frameworks: gitignore
Branching
Git branch
Command |
Description |
$ git branch |
πΏ Show a list of all branches in your local repository. |
$ git branch -a |
πΏ Show all branches in both local and remote repositories. |
$ git branch -r |
πΏ Show only remote branches. |
$ git branch name |
πΏ Creates a new branch called name based on the current commit. |
$ git branch name hash |
πΏ Create a new branch based on a specific commit identified by its hash. |
Git checkout
Command |
Description |
$ git checkout branch-name |
π Switch between branches in a repository. |
$ git checkout -b branch-name |
π Create a new branch and switch to it. |
Git stash
Command |
Description |
$ git stash |
π¦ Stash current work. |
$ git stash save "your message" |
π¦ Saving stashes with a message. |
$ git stash list |
π¦ Check the stored stashes. |
$ git stash apply |
π¦ Re-apply the changes that you just stashed. |
$ git stash show |
π¦ Track the stashes and their changes. |
$ git stash pop |
π¦ Re-apply the previous commits. |
$ git stash drop |
π¦ Delete the most recent stash from the queue. |
$ git stash clear |
π¦ Delete all the available stashes at once. |
$ git stash branch |
π¦ Stash work on a separate branch. |
Merging
Git merge
Command |
Description |
$ git merge name |
π€ Merges branch called name into the current branch, creating a new commit that incorporates both changes. |
$ git merge --abort |
π€ Aborts the merge process and restores the original state of your project, if there are any conflicts or errors during the merge. |
Git rebase
Command |
Description |
$ git rebase |
π Apply a sequence of commits from one branch to another. |
$ git rebase -continue |
π Continue the rebasing process after resolving conflicts manually. |
$ git rebase --skip |
π Skip a commit when rebasing. |
Remote
Command |
Description |
$ git remote |
π Show a list of all remote repositories associated with your local repository. |
$ git remote -v |
π Check the configuration of the remote server. |
$ git remote show name |
π Show information about a specific remote repository called name . |
$ git remote add origin repo-url |
π Add a remote for the repository. |
$ git remote rm |
π Remove a remote connection from the repository. |
$ git remote rename |
π Rename a remote server. |
$ git remote show |
π Show additional information about a particular remote. |
$ git remote set-url name url |
π Change the URL of a remote repository called name to url. |
Pushing Updates
Command |
Description |
$ git push origin master |
π€ Push data to the remote repository. |
$ git push --all |
π€ Push all branches to the default remote repository. |
$ git push --all origin |
π€ Specify the remote repository explicitly. |
$ git push -f |
π€ Force push data to the remote repository. |
Pulling Updates
Git pull
Command |
Description |
$ git pull |
π₯ Pull changes from the default remote repository and branch. |
$ git pull origin master |
π₯ Specify the remote repository and branch explicitly. |
Git fetch
Command |
Description |
$ git fetch <repository URL> |
π₯ Fetch the remote repository. |
$ git fetch |
π₯ Fetch a specific branch. |
$ git fetch --all |
π₯ Fetch all branches simultaneously. |
$ git fetch origin |
π₯ Synchronize the local repository. |
Undo Changes
Git revert
Command |
Description |
$ git revert HEAD |
βͺ Undo the latest commit. |
$ git revert hash |
βͺ Create a new commit that undoes changes made by a specific commit identified by its hash. |
$ git revert branch |
βͺ Undo all commits on a branch. |
Git reset
Command |
Description |
$ git reset --soft hash |
βͺ Reset your current branch to a specific commit identified by its hash, keeping your working directory and staging area unchanged. |
$ git reset --mixed hash |
βͺ Reset your current branch to a specific commit identified by its hash, resetting your staging area to match it, but keeping your working directory unchanged. |
$ git reset --hard hash |
βͺ Reset your current branch to a specific commit identified by its hash, resetting your working directory and staging area to match it, discarding any changes made since then. |
Removing Files
Command |
Description |
$ git rm file |
ποΈ Delete a file from both your working directory and staging area, staging the deletion for the next commit. |
$ git rm --cached file |
ποΈ Delete a file only from the staging area, but not from the working directory. |
Reference